码丁实验室,一站式儿童编程学习产品,寻地方代理合作共赢,微信联系:leon121393608。
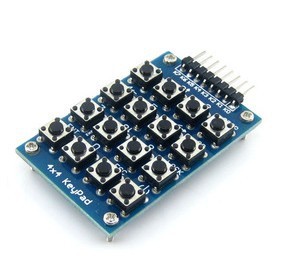
01准备材料
- Arduino Uno 开发板;
- 4*4矩阵键盘
- 8根跳线
02接线
- Keypad Pin R1 –> Arduino Pin 2
- Keypad Pin R2 –> Arduino Pin 3
- Keypad Pin R3 –> Arduino Pin 4
- Keypad Pin R4 –> Arduino Pin 5
- Keypad Pin C1 –> Arduino Pin 6
- Keypad Pin C2 –> Arduino Pin 7
- Keypad Pin C3 –> Arduino Pin 8
- Keypad Pin C4 –> Arduino Pin 9
03所需库文件
-
在Arduino IDE 1.6.2 或者以上版本中,
项目
->加载库
->管理库
中搜索Keypad
,然后安装即可。 -
也可以在这里下载库,然后手动添加到IDE中。
04初始化设置
keypad(makeKeymap(userKeymap), row[], col[], rows, cols)
const byte rows = 4; //four rows
const byte cols = 3; //three columns
char keys[rows][cols] = {
{'1','2','3'},
{'4','5','6'},
{'7','8','9'},
{'#','0','*'}
};
byte rowPins[rows] = {5, 4, 3, 2}; //connect to the row pinouts of the keypad
byte colPins[cols] = {8, 7, 6}; //connect to the column pinouts of the keypad
Keypad keypad = Keypad( makeKeymap(keys), rowPins, colPins, rows, cols );
05常用函数
void begin(makeKeymap(userKeymap))
Initializes the internal keymap to be equal to userKeymap
[See File -> Examples -> Keypad -> Examples -> CustomKeypad]
char waitForKey()
This function will wait forever until someone presses a key. Warning: It blocks all other code until a key is pressed. That means no blinking LED’s, no LCD screen updates, no nothing with the exception of interrupt routines.
char getKey()
Returns the key that is pressed, if any. This function is non-blocking.
KeyState getState()
Returns the current state of any of the keys.
The four states are IDLE, PRESSED, RELEASED and HOLD.
boolean keyStateChanged()
New in version 2.0: Let’s you know when the key has changed from one state to another. For example, instead of just testing for a valid key you can test for when a key was pressed.
setHoldTime(unsigned int time)
Set the amount of milliseconds the user will have to hold a button until the HOLD state is triggered.
setDebounceTime(unsigned int time)
Set the amount of milliseconds the keypad will wait until it accepts a new keypress/keyEvent. This is the “time delay” debounce method.
addEventListener(keypadEvent)
Trigger an event if the keypad is used. You can load an example in the Arduino IDE.
[See File -> Examples -> Keypad -> Examples -> EventSerialKeypad] or see the KeypadEvent Example code.
06示例代码
#include <Keypad.h>
const byte ROWS = 4; //four rows
const byte COLS = 3; //three columns
char keys[ROWS][COLS] = {
{'1','2','3'},
{'4','5','6'},
{'7','8','9'},
{'#','0','*'}
};
byte rowPins[ROWS] = {5, 4, 3, 2}; //connect to the row pinouts of the keypad
byte colPins[COLS] = {8, 7, 6}; //connect to the column pinouts of the keypad
Keypad keypad = Keypad( makeKeymap(keys), rowPins, colPins, ROWS, COLS );
void setup(){
Serial.begin(9600);
}
void loop(){
char key = keypad.getKey();
if (key != NO_KEY){
Serial.println(key);
}
}
07参考文献
http://playground.arduino.cc//Code/Keypad