有人问我其他教练都是教小学生和初中生图形编程,为什么唯独我是拒绝教图形编程,只教文字编程呢?冠冕堂皇的答复不想说,真实答复主要有两点:
1. 我压根就不会图形编程哦!这辈子玩图形编程不超过 1 小时!
2. 我希望我的学生能感觉自己比别人更优越!
有一次小学比赛,对手学生看见我的学生电脑,问他的教练,我们的“程序”怎么那么奇怪,没图的!他的教练说,你有机会读大学的软件编程,你就会知道了!那个学生的表情好像在说:我还玩个毛哦!
这种优越感,或者说为了保持这种优越感,对学生的影响是惊人的!他们会不断追求技术的突破,来保持和对手的距离。
废话不多说,继续翻译:
EV3: Lesson 3 – Python Syntax
EV3:第三课 – Python 语法
3.1 Variables
3.1 变量
Variables store values that can be changed within the programs, simple variable types are:
Boolean: either True or False;
Integer: integers
Float: storing numbers with or without decimal point
String: storing characters
变量是用来在程序内储存一些可改变的数值或字符串,简单的变量类型为:
Boolean: 真假类型,又叫【布尔】类型,数值只能是 True 或 False
Integer: 整数类型
Float: 整数或有小数点的数字
String: 字符串
3.2 Conditional and Loop statements
3.2 条件式或循环语句
if, for, while … etc. Take a look at the following example:
包括 if, for, while 等等,请看以下例子:
#!/usr/bin/env python3
from ev3dev.ev3 import *
from time import sleep, time
import traceback
# It is always a good practice to put codes inside the "try" region, to catch any exceptional bugs of the program
try:
lcd = Screen()
lcd.clear()
# Integer operations
intA = 3
intB = 4
# str(x) means turn x into string format, the following statement will generate an error without the str() function, because a "string" CANNOT ADD an "integer"
lcd.draw.text((5, 5), 'intA + intB = ' + str(intA + intB))
# Boolean, "if" statement, and "pass" statement
bolC = False;
if (bolC == True):
# pass means DO NOTHING, used for occupying spaces for future updates
pass
else:
lcd.draw.text((5, 20), 'bolC is False')
# "For" loop
intCount = 0
# "for intI in range(1,5)" MEANS "for intI = 1 to 4"
for intI in range(1,5):
intCount = intCount + intI
lcd.draw.text((5, 35), 'intCount = ' + str(intCount))
# "While" loop
intCount = 0
intI = 1
while (intI <= 5):
# Statements below this line belong to the "while" loop
intCount = intCount + intI
# intI += 1 is the short form of intI = intI + 1
intI += 1
# Statements above this line belong to the "while" loop
#The following statement DOES NOT belong to the while loop because it has the SAME INDENT as the while loop
lcd.draw.text((5, 50), 'intCount = ' + str(intCount))
# "While" loop with "break"
intCount = 0
intI = 1
while (intI <= 5):
intCount = intCount + intI
intI += 1
if (intCount > 5):
# Means when intCount > 5, leave the whle loop
break
lcd.draw.text((5, 65), 'intCount = ' + str(intCount))
# Display result on LCD Screen
lcd.update()
sleep(10)
except:
# If there is any error, it will be stored in the log file in the same directory
logtime = str(time())
f=open("log" + logtime + ".txt",'a')
traceback.print_exc(file=f)
f.flush()
f.close()
http://www.zephan.top/ev3pythonlessons/lesson3/lesson3_01.py
Upload and run the above program, you’ll see:
上传并运行上面的程序,你会看见:
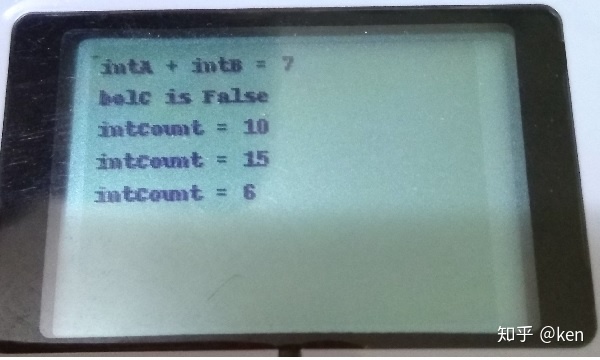
Figure 3.1
3.3 List
3.3 List 【列表】
Sometimes we don’t want to store values in many variables, but store them using one variable name with an index, for example:
有时候我们不希望用很多不同名字的变数名称,去储存很多的变数,我们会用一个变数名称和一个序列号,举例:
#!/usr/bin/env python3
from ev3dev.ev3 import *
from time import sleep, time
import traceback
try:
lcd = Screen()
lcd.clear()
# Declare an Empty List
listNumber = []
# Please note that the "INDEX" of a "LIST" starts from 0
for intI in range(0,5):
listNumber.insert(intI, intI * intI)
# Now display the contents inside listNumber
for intI in range(0,5):
lcd.draw.text((5, intI * 15), str(intI) + " x " + str(intI) + " = " + str(listNumber[intI]))
lcd.update()
sleep(10)
except:
# If there is any error, it will be stored in the log file in the same directory
logtime = str(time())
f=open("log" + logtime + ".txt",'a')
traceback.print_exc(file=f)
f.flush()
f.close()
http://www.zephan.top/ev3pythonlessons/lesson3/lesson3_02.py
The output becomes:
上传并运行上面的程序,你会看见:
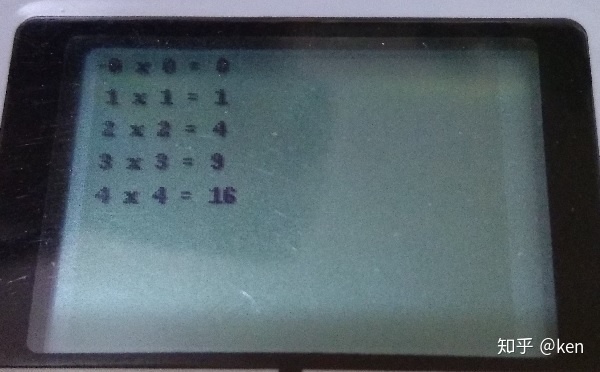
Figure 3.2
3.4 Functions and Global / Local variables
3.4 函数及全局/本地变量
Function: perform repeated jobs.
Local Variable: it can only be used within a particular function
Global Variable: it can be used anywhere in the program
Function 【函数】:用来执行一些重复的工作。
Local Variable【本地变量】:只能用于某一函数内。
Global Variable【全局变量】:在整个程序里都可以使用它。
for example:
举例:
#!/usr/bin/env python3
from ev3dev.ev3 import *
from time import sleep, time
import traceback
# Define function at the beginning of the main program!!!
# Because function cannot be called before the definition
# Which means that you cannot define the below functions at the bottom of this program
def funAddWithReturn(intNo1, intNo2):
intTemp = intNo1 + intNo2
return intTemp
def funAddWithoutReturn(intNo1, intNo2):
global intResult
intResult = intNo1 + intNo2
def funAddWithoutReturnWrong(intNo1, intNo2):
# This function is wrong, it forget to global the variable intResult,
# so after calculating the Addition, the result intResult will be discarded
intResult = intNo1 + intNo2
try:
#The main program starts here
# Declare a global variable
global intResult
intResult = 0
lcd = Screen()
lcd.clear()
# Declare local variable
intA = 3
intB = 5
# The answer for the following statement is 8
lcd.draw.text((5, 5), "intA + intB = " + str(funAddWithReturn(intA, intB)))
# The answer for the following statement is 0
funAddWithoutReturnWrong(intA, intB)
lcd.draw.text((5, 20), "intA + intB = " + str(intResult))
# The answer for the following statement is 8
funAddWithoutReturn(intA, intB)
lcd.draw.text((5, 35), "intA + intB = " + str(intResult))
lcd.update()
sleep(10)
except:
# If there is any error, it will be stored in the log file in the same directory
logtime = str(time())
f=open("log" + logtime + ".txt",'a')
traceback.print_exc(file=f)
f.flush()
f.close()
http://www.zephan.top/ev3pythonlessons/lesson3/lesson3_03.py
The output becomes:
上传并运行上面的程序,你会看见:
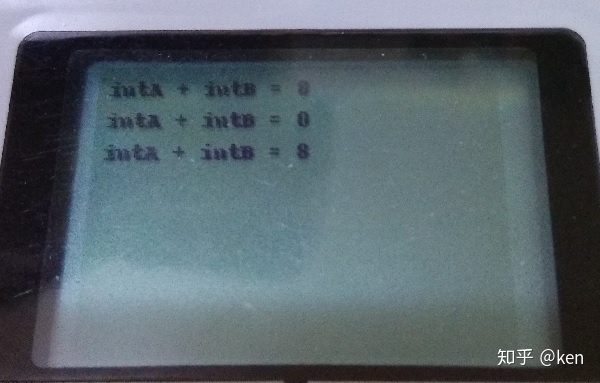
Figure 3.3
The purpose of this website is NOT to learn Python, but learn how to use Python programs to control EV3, for more information about Python, you may want to visit the following web sites:
这个网站的目的不是用来学习 Python 的,而是用来学习如何使用 Python 程序控制 EV3 的,如果你需要更多的 Python 资讯,可以浏览下面的网站:
English Web Site:
英语网站:
https://www.codecademy.com/catalog/language/python
Chinese Web Site:
中文网站:
https://www.w3cschool.cn/python/
In our next lesson, we’ll learn how to store functions in another python program(we call it python modules), so that those functions can be reused in other python projects, and to shorten the length of your main program.
在下一课,我们会学习如何把函数存到另外一个 Python 程序(我们叫 Python 模块),以致这些函数可以被其他的 Python 项目使用(就是不用每次重写),并且可以让你的主程序更短及更易读。
始发于知乎专栏:ken